Python Fast Generate Asymmetric Key
- Python Fast Generate Asymmetric Key Cryptography
- Python Fast Generate Asymmetric Key Algorithm
- Asymmetric Key Example
- Asymmetric Key Algorithm
- Python Fast Generate Asymmetric Key Encryption
- Python Fast Generate Asymmetric Keys
- Python Fast Generate Asymmetric Key Algorithm
- Python Fast Generate Asymmetric Keyboard
- I realize that this question may be borderline bannable because it's asking for suggestions on tools, but it will really help newbies. This online tool allowed me to play around with hashes and to.
- ECC implements all major capabilities of the asymmetric cryptosystems: encryption, signatures and key exchange. The ECC cryptography is considered a natural modern successor of the RSA cryptosystem, because ECC uses smaller keys and signatures than RSA for the same level of security and provides very fast key generation, fast key agreement.
- Oct 30, 2017 How does public-key cryptography work? What is a private key and a public key? Why is asymmetric encryption different from symmetric encryption? I'll explain all of these in plain English!
- Getting a Key
Using the cryptography module in Python, this post will look into methods of generating keys, storing keys and using the asymmetric encryption method RSA to encrypt and decrypt messages and files. We will be using cryptography.hazmat.primitives.asymmetric.rsa to generate keys.
This is a “Hazardous Materials” module. You should ONLY use it if you’re 100% absolutely sure that you know what you’re doing because this module is full of land mines, dragons, and dinosaurs with laser guns. Unlike symmetric cryptography, where the key is typically just a random series of bytes, RSA keys have a complex internal structure with specific mathematical properties. Cryptography.hazmat.primitives.asymmetric.rsa.generateprivatekey (publicexponent, keysize, backend) source ¶. AsyncSSH: Asynchronous SSH for Python. The server’s host key is checked against the user’s SSH knownhosts file and the connection will fail if there’s no entry for localhost there or if the key doesn’t match. Note that it uses the createprocess method rather than the run method. This starts the process but doesn’t wait for it. I'm attempting to write a script to generate SSH Identity key pairs for me. How to generate SSH key pairs with Python. Hazmat.primitives.asymmetric import rsa.
Installing cryptography
Since Python does not come with anything that can encrypt files, we will need to use a third party module.
PyCrypto is quite popular but since it does not offer built wheels, if you don't have Microsoft Visual C++ Build Tools installed, you will be told to install it. Instead of installing extra tools just to build this, I will be using the cryptography module. To install this, execute:
To make sure it installed correctly, open IDLE and execute:
Python Fast Generate Asymmetric Key Cryptography
Wondershare dr fone serial key generator download without. If no errors appeared it has been installed correctly.
What is Asymmetric Encryption?
If you read my article on Encryption and Decryption in Python, you will see that I only used one key to encrypt and decrypt.
Asymmetric encryption uses two keys - a private key and a public keys. Public keys are given out for anyone to use, you make them public information. Anyone can encrypt data with your public key and then only those with the private key can decrypt the message. This also works the other way around but it is convention to keep your private key secret.
Getting a Key
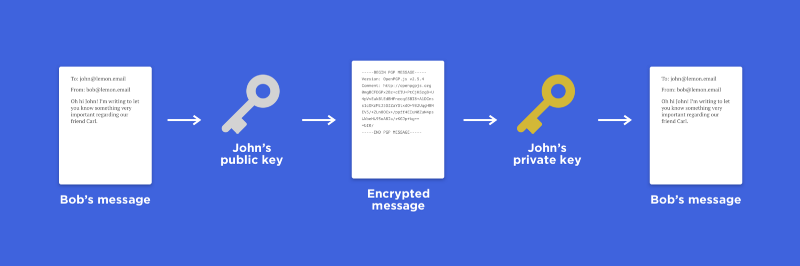
To generate the two keys, we can call rsa.generate_private_key with some general parameters. The public key will be found in the object that holds the creation of the private key.
Storing Keys
Python Fast Generate Asymmetric Key Algorithm
To store the keys in a file, they first need to be serialized and then written to a file. To store the private key, we need to use the following.
You can password protect the contents of this file using this top key serialization example.
To store the public key, we need to use a slightly modified version.
Remember that public and private keys are different so you will have to use these methods for each key.
Reading Keys
To get the keys out of the files, we need to read each file and then load them. To read the private key, use the following.
If you store the key with a password, set password to what you used.
The variable private_key will now have the private key. To read the public key, we need to use a slightly modified version.
The variable public_key will now have the public key.
Asymmetric Key Example
Encrypting
Due to how asymmetric encryption algorithms like RSA work, encrypting with either one is fine, you just will need to use the other to decrypt. Applying a bit of logic to this can create some useful scenarios like signining and verification. For this example I will assume that you keep both keys safe and don't release them since this example is only for local encryption (can be applied to wider though when keys are exchanged).
This means you can use either key but I will demonstrate using the public key to encrypt, this will mean anyone with the private key can decrypt the message.
Decrypting
Assuming that the public key was used to encrypt, we can use the private key to decrypt.
Asymmetric Key Algorithm
Demonstration
Python Fast Generate Asymmetric Key Encryption
To show this in action, here is a properly constructed example.
Encrypting and Decrypting Files
Python Fast Generate Asymmetric Keys
To encrypt and decrypt files, you will need to use read and write binary when opening files. You can simply substitute the values I previous used for message with the contents of a file. For example:
Python Fast Generate Asymmetric Key Algorithm
Using the variable messageWarcraft 3 roc key generator. you can then encrypt it. To store, you can use the general Python method when encryption returns bytes.
Python Fast Generate Asymmetric Keyboard
Now to decrypt you can easily read the data from test.encrypted like the first bit of code in this section, decrypt it and then write it back out to test.txt using the second bit of code in this section.